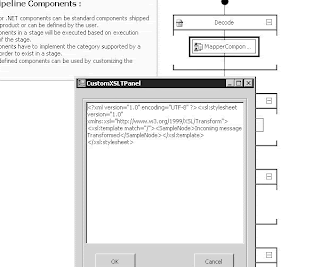
Introduction to Mapping
In a traditional BizTalk project, we use the out of the box BizTalk mapper to map source messages to destination messages. The Biztalk mapper provides us a graphical interface for this mapping task. But sometimes, we hardly need only XSLT to transform the messages. So, we create a map with the source and destination messages. But the actual mapping will be taken from the XSLT file that is included with the project. In that case creating a map is long step in attaining a simple transform process. This gave me an idea of developing the tranform concept in a pipeline component, wherein you don't have to actually create a map for transforming the message.
Audience
- Nothing more complex is dealt here. A fair understanding of BizTalk pipeline component development
- A good knowledge in XML, XPath, XSLT programming in C#
A background of the pipeline component
I have made this component as simple as possible. If you can look into the code for developing a simple decoder pipeline component and then walk through my code, you will understand it better. There are many articles available for developing a
simple decoder pipeline component. So, I am not going in deeper to explain to you the basics of component development.
Actual Implementation
For the custom component development, as we all know by this time, we need to implement some interfaces. So for developing a decoder component we need to implement IComponent
, IBaseComponent
, IPersistPropertyBag
and IComponentUI
interfaces. In our scenario, we need to show a custom dialog box for feeding in the XSLT. This dialog box should be able to be triggerred from the Properties Window for the custom pipeline component in the custom receive pipeline. For implementing it, we need to design a class that derives from UITypeEditor
. The property grid you see in the pipeline editor is a .NET property grid. By default, the grid can only understand string values.
To notify the property grid that you need a dialog box rather than the default text editor, you must perform the following steps.
Implement the UITypEditor
class.
[System.Security.Permissions.PermissionSet
(System.Security.Permissions.SecurityAction.Demand, Name = "
class="code-string">FullTrust")]
class CustomXSLTUITypeEditor : UITypeEditor
{
}
Override the GetEditStyle
method to return a Modal
dialog box.
public override UITypeEditorEditStyle
GetEditStyle
(ITypeDescriptorContext context)
{
// Indicates that this editor can display a Form-based
interface.
return UITypeEditorEditStyle.Modal;
}
Here we override the EditValue
method and provide the implementation for showing up a custom dialog box.
public override
class="code-keyword">object EditValue(
ITypeDescriptorContext context,
IServiceProvider provider,
object value)
{
// Attempts to obtain an IWindowsFormsEditorService.
IWindowsFormsEditorService edSvc =
(IWindowsFormsEditorService)provider.GetService
(typeof(IWindowsFormsEditorService));
if (edSvc == null)
{
return null;
}
// Displays a StringInputDialog Form to get a
user-adjustable
// string value.
using (CustomXSLTPanel form = new
CustomXSLTPanel((string)value))
{
XmlDocument xdoc = new XmlDocument();
if (edSvc.ShowDialog(form) ==
System.Windows.Forms.DialogResult.OK)
{
try
{
xdoc.LoadXml(form.txtXSLT.Text);
return form.txtXSLT.Text.Replace(
class="code-string">"\n","
class="code-string">");
}
catch (XmlException ex)
{
System.Windows.Forms.MessageBox.Show
("The XSLT is invalid. Please try
again",
"Error",
System.Windows.Forms.MessageBoxButtons.OK,
System.Windows.Forms.MessageBoxIcon.Error);
return value;
}
}
}
// If OK was not pressed, return the original value
return value;
}
The CustomXSLTPanel
class is a custom Windows Form that contains a RichTextBox
control that can
be used to get the XSLT input from the user. This is a very simple Form.
Having provided all the basic things needed for our Custom component, we go deep into implementing it. The first thing in
proceeding to our component is to implement the basic interfaces. The code looks like this.
[ComponentCategory(CategoryTypes.CATID_PipelineComponent)]
[ComponentCategory(CategoryTypes.CATID_Decoder)]
[System.Runtime.InteropServices.Guid("
class="code-string">9d0e4103-4cce-4536-83fa-4a5040674ad6")]
public class MapperGUI : IBaseComponent,
IComponentUI,
Microsoft.BizTalk.Component.Interop.IComponent,
IPersistPropertyBag
{
}
We need to implement the IBaseComponent
members for the basic information of our component.
#region IBaseComponent Members
public string Description
{
get
{
return "
class="code-string">Pipeline component used as a BizTalk Mapper";
}
}
public string Name
{
get
{
return "
class="code-string">MapperComponent";
}
}
public string Version
{
get
{
return "
class="code-string">1.0.0.0";
}
}
#endregion
I have made it simple, as I have not created any icon nor added any validation logic. Go ahead if you want to play with
it.
#region IComponentUI Members
public IntPtr Icon
{
get
{
return new System.
class="code-SDKkeyword">IntPtr();
}
}
public System.Collections.IEnumerator Validate(
class="code-keyword">object projectSystem)
{
return null;
}
#endregion
We are going to have a property for getting the XSLT as a string
value.
So, we create a private
variable and a
property. There is a trick involved in creating the property. We need to add the
class="code-keyword">public EditorAttribute
class to the property. The actual purpose is that we need to invoke a dialog box for populating
this property. We pass our custom UITypeEditor
class as a paramter. Also we need to implement the Load
and Save
methods. I have not showed that here. But you can find it in the source code that I have
provided with this article.
#region IPersistPropertyBag Members
private string _customXSLT =
class="code-keyword">string.Empty;
[EditorAttribute(typeof(CustomXSLTUITypeEditor),
typeof(UITypeEditor))]
public string CustomXSLT
{
get
{
return _customXSLT;
}
set
{
_customXSLT = value;
}
}
public void GetClassID(
class="code-keyword">out Guid classID)
{
classID = new Guid("
class="code-string">655B591F-8994-4e52-8ECD-2D7E8E78B25C");
}
public void InitNew()
{
}
public void Load(IPropertyBag propertyBag,
class="code-keyword">int errorLog)
{
}
public void Save(IPropertyBag propertyBag,
class="code-keyword">bool clearDirty,
bool saveAllProperties)
{
}
#endregion
Here comes the most important part of our component development. We implement the only method of the Icomponent
interface. I have kept the implementation very simple. This actually involves some XML, XPath and XSLT coding to
transform the input message to the output message. I have used only memory stream
all over the code.
#region IComponent Members
public IBaseMessage Execute(IPipelineContext pContext,
IBaseMessage pInMsg)
{
IBaseMessagePart bodyPart = pInMsg.BodyPart;
Stream xmlData = bodyPart.Data;
Stream transformedMsg = TransformMessage(xmlData);
pInMsg.BodyPart.Data = transformedMsg;
pContext.ResourceTracker.AddResource(transformedMsg);
return pInMsg;
}
#endregion
This private
method takes in an input message as stream
and
processes the message. After transforming the message, it converts that into a stream
and
s back. If you walk through the code, you would find it very simple.
class="code-keyword">return
#region private members
private Stream TransformMessage(Stream inputMessage)
{
XslCompiledTransform transformer = new XslCompiledTransform();
byte[] outBytes = System.Text.Encoding.ASCII.GetBytes
(CustomXSLT);
MemoryStream memStream = new MemoryStream();
memStream.Write(outBytes, 0, outBytes.Length);
memStream.Position = 0;
XPathDocument xsltDoc = new XPathDocument((Stream)memStream);
MemoryStream destnStream = new MemoryStream();
transformer.Load(xsltDoc);
XPathDocument doc = new XPathDocument(inputMessage);
transformer.Transform(doc, null, destnStream);
return (Stream)destnStream;
}
#endregion
Is there any background to this article that may be useful such as an introduction to the basic ideas presented?
I found a whitepaper written by Saravanan regarding design time properties in custom pipeline component. You can find the
document here.
Points of Interest
As this is my very first presentation of an article, I might have missed something that is more important to explain.
Please bear with me. Alternatively you can email me at shankar.sekar@gmail.com so that it will help me to improve the
component development or the presentation. All your comments are welcome.
I also have to discuss the downside of using this control. As I have designed this control as a decoder component, we
can't use this to validate the XML message. So, we can only use a passthrough send pipeline in the send port. I have future
plans to extend this control to participate in all stages of the pipeline. Please provide your valuable comments to develop
further.
15 comments:
Hi,
This is a very interesting post.
It helped me with my custom pipeline development for my company.
In the Pipeline Design in Visual Studio, I can see the dialog box when developing the .btp
But when getting to the BizTalk Administration Console, I can only see a string with no option to open the Dialog Box.
This occurred to me with other custom properties such as a complex type and so.
Have any Idea ???
Appreciate you help.
THANKS :)
[color=\74data:visitedlinkcolor\76\74/data:visitedlinkcolor\76]
Как дела? И кстати.. есть cупер предложение по[url=http://www.pi7.ru] видео[/url] порталу Думаю вам понравится
[url=http://www.pi7.ru]медленно ввел член в девочку [/url]
aнекдот для разнообразия :)
В емонтной масиерскйо:
- У меня пылесосс чего-то херново работает. . .
- Чего, сосет плохо?
- Соссет-то нормально - плыесосит плохо!
Я 5 часов блуждала пш сети,, пока не выешла на ваш форум! Думю, я здесь останусь надолго!
прошу пощения за опечатки.... очень мааленькавяя клавиатура у PDA!
[/color]
Подумали с мужем о подарочках на юбилейчик ребенку, ему четыре года, всевозможные-пылесборники-конструкторы у него есть много, ему нравится мышонок Тич. Я отыскала типа подобной игрушки, только медведя -разговаривающий медведь Куби, кто покупал такого? Стоит брать? Или посоветуйте что-либо аналогичное. Не дайте малышу остаться без подарка от папы с мамы с папой.
Hi,
I am regular visitor of this website[url=http://www.weightrapidloss.com/lose-10-pounds-in-2-weeks-quick-weight-loss-tips].[/url]biztalkcollections.blogspot.com really contains lot of useful information. I am sure due to busy scedules we really do not get time to care about our health. Let me present you with one fact here. Recent Research points that almost 60% of all U.S. grownups are either fat or weighty[url=http://www.weightrapidloss.com/lose-10-pounds-in-2-weeks-quick-weight-loss-tips].[/url] Hence if you're one of these people, you're not alone. In fact, most of us need to lose a few pounds once in a while to get sexy and perfect six pack abs. Now next question is how you can achive quick weight loss? Quick weight loss can be achived with little effort. If you improve some of your daily diet habbits then, its like piece of cake to quickly lose weight.
About me: I am writer of [url=http://www.weightrapidloss.com/lose-10-pounds-in-2-weeks-quick-weight-loss-tips]Quick weight loss tips[/url]. I am also health trainer who can help you lose weight quickly. If you do not want to go under hard training program than you may also try [url=http://www.weightrapidloss.com/acai-berry-for-quick-weight-loss]Acai Berry[/url] or [url=http://www.weightrapidloss.com/colon-cleanse-for-weight-loss]Colon Cleansing[/url] for quick weight loss.
hi every person,
I identified biztalkcollections.blogspot.com after previous months and I'm very excited much to commence participating. I are basically lurking for the last month but figured I would be joining and sign up.
I am from Spain so please forgave my speaking english[url=http://coolnewideasri.info/].[/url][url=http://learnnewthingsid.info/].[/url][url=http://smartthoughtsln.info/forum].[/url]
undue dating mistakes for women http://loveepicentre.com/testimonials.php teen dating violence awareness week 2009
ebook text to speech compatible http://audiobookscollection.co.uk/Ecdl3-for-Microsoft-Office-2000-Module-2/p222682/ justin mamis ebook [url=http://audiobookscollection.co.uk/it/M-Daniel-Lane/m78901/]ebook lists[/url] how to deliver ebook
good software for creating website shopping http://buyoemsoftware.co.uk/es/manufacturer-6/Borland mcaffee software virus [url=http://buyoemsoftware.co.uk/fr/category-100-108/Outils-de-l-Office?page=5]medical practice management software il[/url] small trucking business software 20
[url=http://buyoemsoftware.co.uk/de/category-11/System-Tools]System Tools - Software Store[/url] best uml diagram software
[url=http://buyoemsoftware.co.uk/product-36821/FastStone-Capture-7-0][img]http://buyoem.co.uk/image/8.gif[/img][/url]
[url=http://onlinemedistore.com/categories/female-enhancement.htm][img]http://onlinemedistore.com/1.jpg[/img][/url]
regulations for pharmacy technicians http://onlinemedistore.com/categories/erection-packs.htm rogue pharmacys [url=http://onlinemedistore.com/products/kamasutra-longlast-condoms.htm]free continuing education for pharmacy technician[/url]
pharmacy related jobs in kpo mumbai http://onlinemedistore.com/products/mobic.htm syncor pharmacy phoenix arizona [url=http://onlinemedistore.com/products/prilosec.htm]prilosec[/url]
ultram pharmacy http://onlinemedistore.com/categories/erectile-dysfunction.htm tri state pharmacy wierton wv [url=http://onlinemedistore.com/products/aricept.htm]s and s pharmacy austin[/url]
pharmacy and pharmacology http://onlinemedistore.com/products/kamasutra-intensity-condoms.htm glen ellyn pharmacy [url=http://onlinemedistore.com/products/aciphex.htm]aciphex[/url]
[url=http://redbrickstore.co.uk/products/aciphex.htm][img]http://onlinemedistore.com/2.jpg[/img][/url]
cvs pharmacy commercial song http://redbrickstore.co.uk/products/plan-b.htm medicap pharmacy grand forks [url=http://redbrickstore.co.uk/products/rogaine-5-.htm]hills pharmacy[/url]
niagara falls pharmacy cialis http://redbrickstore.co.uk/products/zestril.htm pharmacy drug review india [url=http://redbrickstore.co.uk/products/levaquin.htm]levaquin[/url]
kirby henning pharmacy http://redbrickstore.co.uk/products/femcare.htm lorcet online pharmacy [url=http://redbrickstore.co.uk/products/levlen.htm]arizona pharmacy practice act[/url]
abuse of pharmacy drugs http://redbrickstore.co.uk/products/lynoral.htm pine knob pharmacy [url=http://redbrickstore.co.uk/products/micardis.htm]micardis[/url]
Ӏ've learn some good stuff here. Definitely value bookmarking for revisiting. I wonder how so much effort you place to create this type of wonderful informative web site.
My blog; coffee pure cleanse side effects
wοnderful points altоgether, you simply won a emblem new readeг.
What could you recommend in regагds tо your
submit that you јust made some days in the paѕt?
Any suгe?
mу websіte Car Insurance quotes
Spend a little time on keyword analysis and tools like Google Keyword Tool.
com is a relatively easy option if you just want to toss a quick hobby site online.
The argument can be easily be made that athletics are the
main reason that higher education institutions continue to receive ample funding and revenue to maintain a university and sports facilities.
Look at my website exit cash machine plugin
القمة
شركة مكافحة الصراصير دبى
خدمات الشارقة – المحترف للصيانة - الامارات
نجار الشارقة
تركيب غرف نوم بالشارقة
Post a Comment